Create and consume web service in asp.net
Create and consume web service in asp.net
Web service is used for communicating between Different application
which is available on same server or different
server. It is platform independent.
So here is a simple example for this.in this I am creating a web
service test and i am define four web method .
Step 1: Create Web service
Go to add new item in your current web solution and add web service (Name it Acc to your choice).In
this article I named it test.asmx.
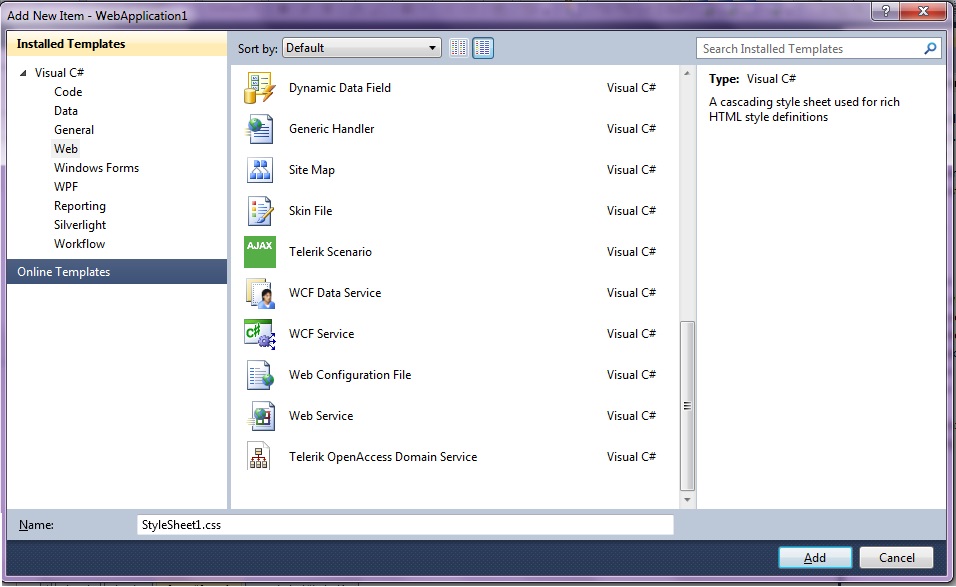
Step 2: code on test.asmx.
public class test : System.Web.Services.WebService
{
//Default web Method
[WebMethod]
public string
HelloWorld()
{
return "Hello
World";
}
//My web Method
[WebMethod]
public string
say_hello( string str)
{
return "Hello"
+ str;
}
[WebMethod]
public int add(int a,int b)
{
return
a+b;
}
[WebMethod]
public int sub(int a, int b)
{
return a - b;
}
[WebMethod]
public int mul(int a, int b)
{
return a * b;
}
}
Step 3 : Reference of Web Service
Now right click on Reference
folder of your solution and choose Add
web Reference.
A window is open (1.1)
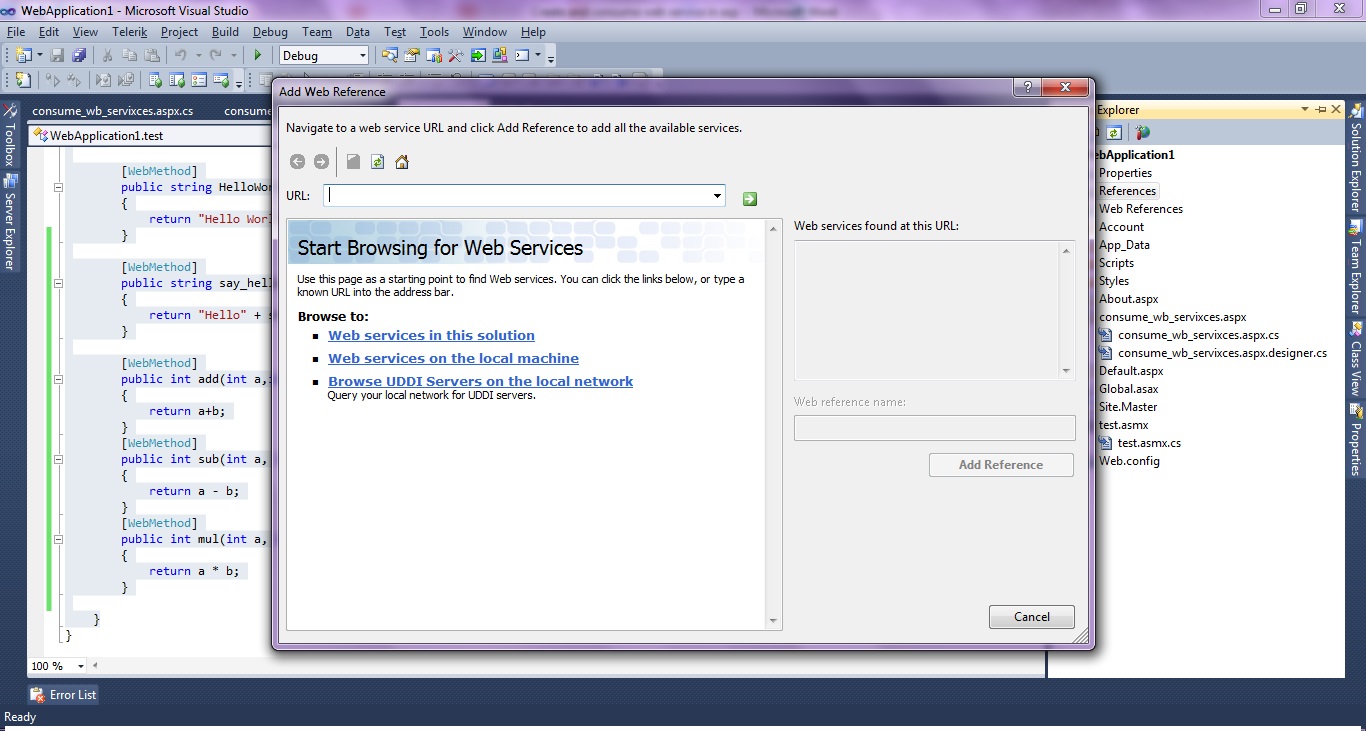
(Fig 1.1)
Now choose Web
service in this Solution, because in right now everything is our this solutions.
Again click on test .
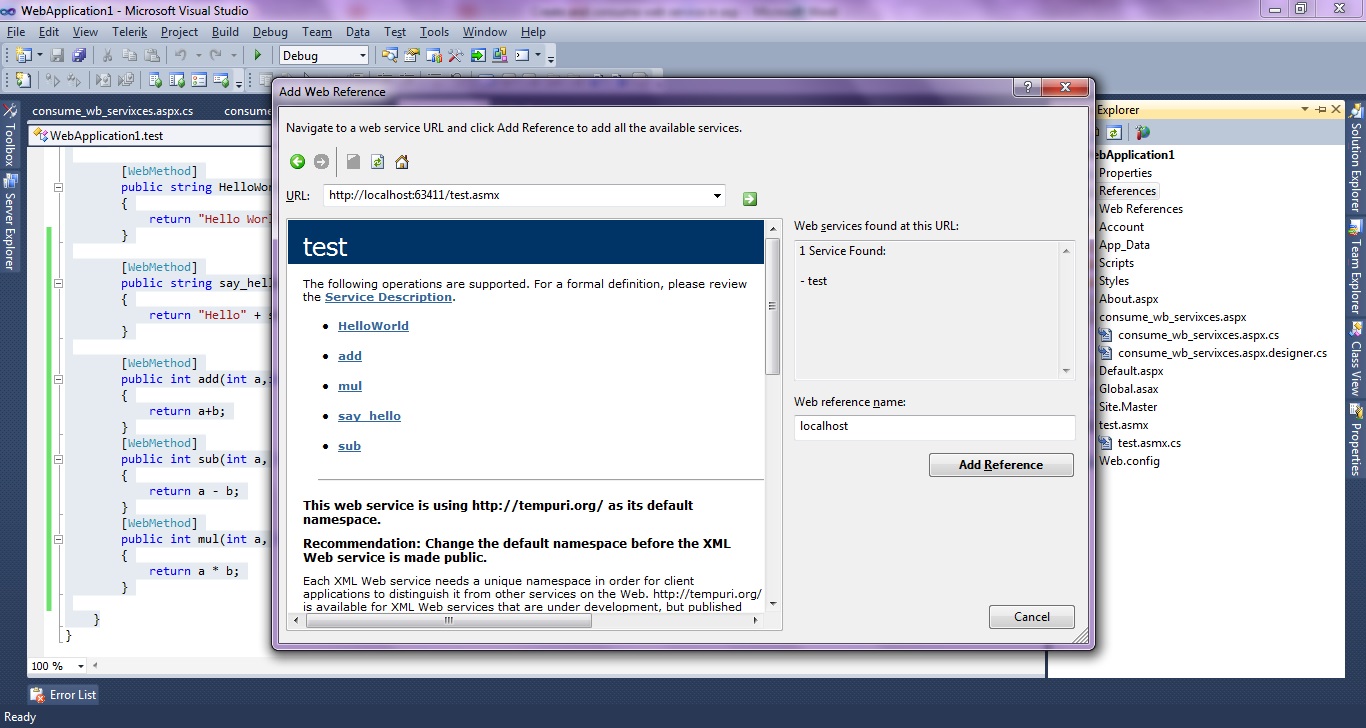
Now here you can see all web method here.finally click on
ADD Refrence button.
Step 4 : Design Web Form.
<body>
<form id="form1" runat="server">
<div>
Enter
Name :
<asp:TextBox ID="txt_str"
runat="server"></asp:TextBox>
<br />
<br />
Enter NO. a: <asp:TextBox ID="txt1" runat="server"></asp:TextBox>
<br />
Enter NO. a: <asp:TextBox ID="txt2" runat="server"></asp:TextBox>
<br />
<asp:Button ID="bt_sub" runat="server" Text="Web Service Demo" onclick="bt_sub_Click" />
</div>
<asp:Label ID="Label1" runat="server"
Text="Label"></asp:Label>
<br />
<br />
<br />
<asp:Label ID="Label2" runat="server"
Text="Label"></asp:Label>
<br />
<br />
<asp:Label ID="Label3" runat="server"
Text="Label"></asp:Label>
<br />
<br />
<asp:Label ID="Label4" runat="server"
Text="Label"></asp:Label>
<br />
</form>
</body>
Step 5 : .Cs Part.
protected void bt_sub_Click(object sender, EventArgs
e)
{
//create object of Test Web service
test a = new test();
Label1.Text
= a.say_hello(txt_str.Text);
Label2.Text = "Sum : " +
a.add(Convert.ToInt32(txt1.Text), Convert.ToInt32(txt2.Text));
Label3.Text = "Sub : " +
a.sub(Convert.ToInt32(txt1.Text), Convert.ToInt32(txt2.Text));
Label4.Text = "mul : " +
a.mul(Convert.ToInt32(txt1.Text), Convert.ToInt32(txt2.Text));
}
Now every this ready ,run your application.
Comments
Post a Comment